Azure Resource Manager (ARM) template is a JavaScript Object Notation (JSON) file that defines the infrastructure and configuration for Azure resources. There are several ways to find the template for the resources. I usually go to the following places:
- Microsoft Docs Azure Template References
- Azure QuickStart template in GitHub
- Azure REST API reference
- Azure Portal deployed a resource template
To find the ARM template for a configured resource in Azure, I can check it in the Export template in the resource on the portal. For example, below is the ARM template for a Network Watcher resource group. I then copy the code and tweak the parameters to a new ARM template for the automation of a new subscription.
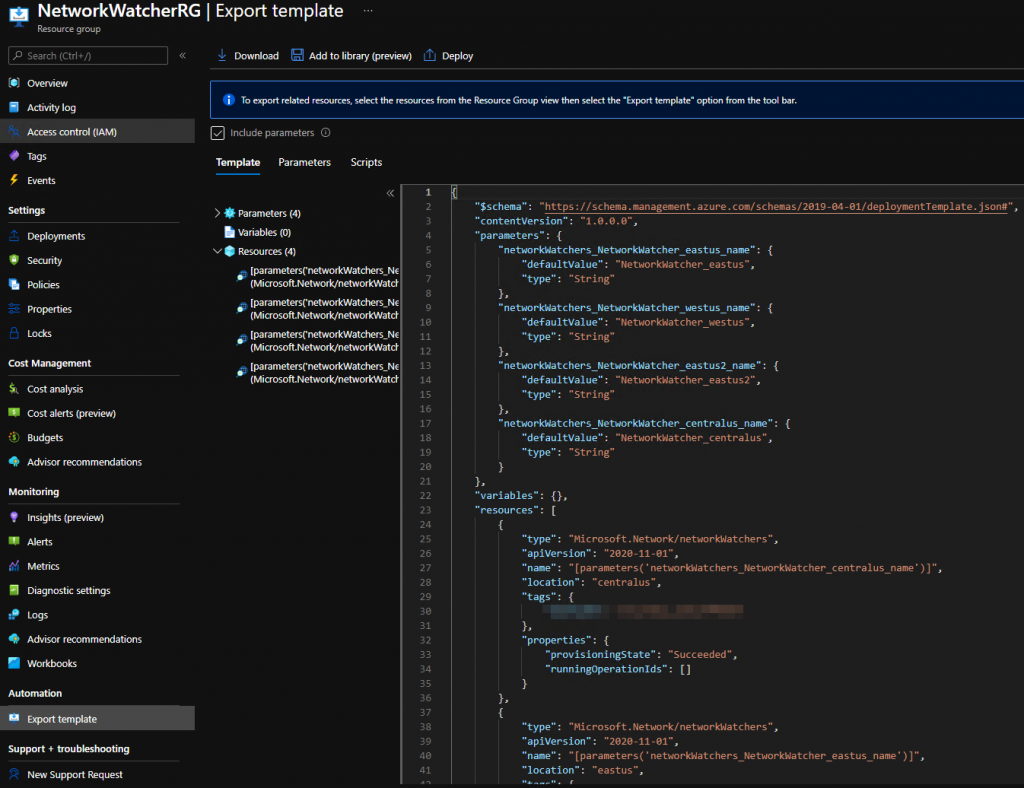
However, there is no Export template option in the Security Center. So I need to find it in another way. Fortunately, I found them under the Security in the Microsoft Docs Azure Template References. Microsoft uses different names for the configurations. For example, the Azure Defender setting is on the Pricings so I decided to document it here for reference. These settings are in the Security Center -> Management -> Pricing & settings. The ARM template will need to be deployed to the subscription level.
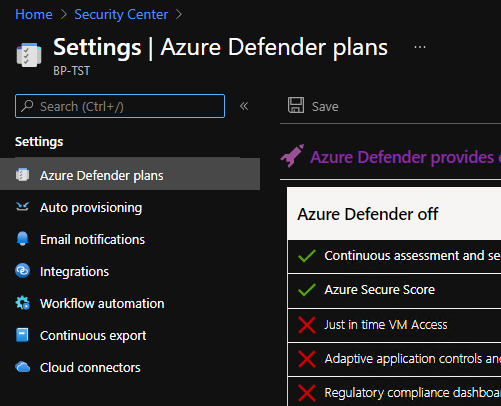
Azure Defender plans
The ARM template will enable the Azure Defender on the resources.
{
"$schema": "https://schema.management.azure.com/schemas/2018-05-01/subscriptionDeploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"virtualMachineTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specifiy whether you want to enable Standard tier for Virtual Machine resource type"
}
},
"appServiceTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Azure App Service resource type"
}
},
"paasSQLServiceTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for PaaS SQL Service resource type"
}
},
"sqlServerOnVmTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for SQL Server on VM resource type"
}
},
"storageAccountTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Storage Account resource type"
}
},
"kubernetesServiceTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Kubernetes service resource type"
}
},
"containerRegistryTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Container Registry resource type"
}
},
"keyvaultTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Key Vault resource type"
}
},
"ArmTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for Resource Manager resource type"
}
},
"DnsTier": {
"type": "string",
"defaultValue": "Standard",
"allowedValues": [
"Standard",
"Free"
],
"metadata": {
"description": "Specify whether you want to enable Standard tier for DNS resource type"
}
}
},
"functions": [],
"variables": {},
"resources": [
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "VirtualMachines",
"properties": {
"pricingTier": "[parameters('virtualMachineTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "AppServices",
"dependsOn": [
"[concat('Microsoft.Security/pricings/VirtualMachines')]"
],
"properties": {
"pricingTier": "[parameters('appServiceTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "SqlServers",
"dependsOn": [
"[concat('Microsoft.Security/pricings/AppServices')]"
],
"properties": {
"pricingTier": "[parameters('paasSQLServiceTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "SqlServerVirtualMachines",
"dependsOn": [
"[concat('Microsoft.Security/pricings/SqlServers')]"
],
"properties": {
"pricingTier": "[parameters('sqlServerOnVmTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "StorageAccounts",
"dependsOn": [
"[concat('Microsoft.Security/pricings/SqlServerVirtualMachines')]"
],
"properties": {
"pricingTier": "[parameters('storageAccountTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "KubernetesService",
"dependsOn": [
"[concat('Microsoft.Security/pricings/StorageAccounts')]"
],
"properties": {
"pricingTier": "[parameters('kubernetesServiceTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "ContainerRegistry",
"dependsOn": [
"[concat('Microsoft.Security/pricings/KubernetesService')]"
],
"properties": {
"pricingTier": "[parameters('containerRegistryTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "KeyVaults",
"dependsOn": [
"[concat('Microsoft.Security/pricings/ContainerRegistry')]"
],
"properties": {
"pricingTier": "[parameters('keyvaultTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "Arm",
"properties": {
"pricingTier": "[parameters('ArmTier')]"
}
},
{
"type": "Microsoft.Security/pricings",
"apiVersion": "2018-06-01",
"name": "Dns",
"properties": {
"pricingTier": "[parameters('DnsTier')]"
}
}
],
"outputs": {}
}
Auto provisioning
The ARM template will enable the Log Analytics agent for Azure VMs.
{
"$schema": "https://schema.management.azure.com/schemas/2018-05-01/subscriptionDeploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"autoProvision": {
"type": "string",
"defaultValue":"On",
"allowedValues": [
"On",
"Off"
]
}
},
"functions": [],
"variables": {},
"resources": [
{
"name": "default",
"type": "Microsoft.Security/autoProvisioningSettings",
"apiVersion": "2017-08-01-preview",
"properties": {
"autoProvision": "[parameters('autoProvision')]"
}
}
],
"outputs": {}
}
Email notifications
The ARM template configures the Email recipients and the Notifications types. Change the default value of the additionalEmailAddress and the minialSeverity to yours.
{
"$schema": "https://schema.management.azure.com/schemas/2018-05-01/subscriptionDeploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"additionalEmailAddresses": {
"type": "string",
"defaultValue":"[email protected]"
},
"minimalSeverity": {
"type": "string",
"defaultValue":"Medium"
},
"notificationRole": {
"type": "string",
"defaultValue": "Owner"
}
},
"functions": [],
"variables": {},
"resources": [
{
"name": "default",
"type": "Microsoft.Security/securityContacts",
"apiVersion": "2020-01-01-preview",
"properties": {
"emails": "[parameters('additionalEmailAddresses')]",
"alertNotifications": {
"state": "On",
"minimalSeverity": "[parameters('minimalSeverity')]"
},
"notificationsByRole": {
"state": "On",
"roles": [
"[parameters('notificationRole')]"
]
}
}
}
],
"outputs": {}
}
Continuous export to the Event hub
This one is a bit complex. You will need to provide the Event hub namespace, Event Hub name, and Event hub policy name in the parameters.
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"namespaces_Security_EHN_name": {
"defaultValue": "your Event hub namespace",
"type": "String"
},
"eventhub_security_name": {
"defaultValue": "your Event Hub Name",
"type": "string"
},
"authorizationRulesName": {
"type": "string",
"defaultValue": "your Event hub policy name"
},
"eventHubDetails": {
"type": "String",
"defaultValue": "[resourceId('Microsoft.EventHub/namespaces/eventhubs/authorizationRules', parameters('namespaces_Security_EHN_name'), parameters('eventhub_security_name'),parameters('authorizationRulesName'))]",
"metadata": {
"displayName": "Event Hub details",
"description": "The Event Hub details of where the data should be exported to: Subscription, Event Hub Namespace, Event Hub, and Authorizations rules with 'Send' claim.",
"strongType": "Microsoft.EventHub/namespaces/eventhubs/authorizationrules",
"assignPermissions": true
}
}
},
"functions": [],
"variables": {
},
"resources": [
{
"tags": {},
"apiVersion": "2019-01-01-preview",
"location": "centralus",
"name": "exportToEventHub",
"type": "Microsoft.Security/automations",
"dependsOn": [],
"properties": {
"description": "Export Azure Security Center data to Event Hub",
"isEnabled": true,
"scopes": [
{
"description": "scope for subscription",
"scopePath": "[subscription().id]"
}
],
"sources": [
{
"eventSource": "Alerts",
"ruleSets": [
{
"rules": [
{
"propertyJPath": "Severity",
"propertyType": "String",
"expectedValue": "medium",
"operator": "Equals"
}
]
},
{
"rules": [
{
"propertyJPath": "Severity",
"propertyType": "String",
"expectedValue": "high",
"operator": "Equals"
}
]
}
]
}
],
"actions": [
{
"sasPolicyName": "[parameters('authorizationRulesName')]",
"actionType": "EventHub",
"eventHubResourceId": "[concat(subscription().Id,'/resourcegroups/',resourceGroup().name,'/providers/microsoft.eventhub/namespaces/',parameters('namespaces_Security_EHN_name'),'/eventhubs/',parameters('eventhub_security_name'))]",
"connectionString": "[listkeys(parameters('eventHubDetails'),'2017-04-01').primaryConnectionString]"
}
]
}
}
],
"outputs": {}
}
Continous export to the Log Analytics workspace
The easy way is to find the workspace resource ID and put it in the “workspaceResouceId”. For example, I created a test Log Analytics workspace for tracking the Secure Score. You can find the Resouce ID by clicking the JSON view in the Overview of the Log Analytics workspace in the Azure portal.
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {},
"functions": [],
"variables": {},
"resources": [
{
"name": "ExportToWorkspace",
"apiVersion": "2019-01-01-preview",
"type": "Microsoft.Security/automations",
"location": "centralus",
"properties": {
"description": "",
"isEnabled": true,
"scopes": [
{
"description": "scope for subscription",
"scopePath": "[subscription().id]"
}
],
"sources": [
{
"eventSource": "Assessments",
"ruleSets": [
{
"rules": [
{
"propertyJPath": "type",
"propertyType": "String",
"expectedValue": "Microsoft.Security/assessments",
"operator": "Contains"
}
]
}
]
},
{
"eventSource": "SubAssessments"
},
{
"eventSource": "SecureScores"
},
{
"eventSource": "SecureScoresSnapshot"
},
{
"eventSource": "SecureScoreControls"
},
{
"eventSource": "SecureScoreControlsSnapshot"
}
],
"actions": [
{
"workspaceResourceId": "/subscriptions/0287117d-2ebc-4227-bc08-55a204302bb4/resourcegroups/my_sc_workbooks/providers/microsoft.operationalinsights/workspaces/my-sc-secure-score-log",
"actionType": "Workspace"
}
]
}
}
],
"outputs": {}
}